JAVA Chat App - OKok
OKok chat
OKok chat is a simple chat application developed using java,
it is meant for "one on one" chat between two people only,It uses java's "java.net" for communicating through sockets.
In this post i'll be posting both the code and HOW TO'S of running the application
PART 1( The application)
#Step 1:
Download the application
Note:
- If two people (ONE and TWO) want to chat with each other then ONE should download Server.jar and TWO should download Client.jar(or vice versa).
- In short if person 1 has server.jar then person 2 should have client.jar
- Also BOTH need to download "jarfix.exe"
You can download the respective file here
1: Server.jar ( 7.1 KB )
2: Client.jar (7 KB)
3: jarfix.exe (63.8 KB)
Things you need to know:
- "IP Address" of the person having Server.jar (click here to find your ip)
- Port no of Server.jar,person having Server.jar needs to choose a port no (any number greater than 1024 and less than 65535, for example: 9090)
- The person having the client.jar should be told the IP Address and port explicitly
#Step 2:
Note: By now the person having "client.jar" should know IP Address and port of server
1: Double click on jarfix.exe (why ? what is this ?) and follow instructions.
2: The individual having Server.jar should double click it, something like the image shown below will appear
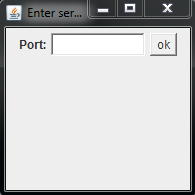
3: Enter a port no (any number greater than 1024 and less than 65535, for example: 9090) and remember it
also the other person or client should be told this number
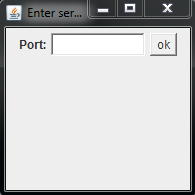
3: Enter a port no (any number greater than 1024 and less than 65535, for example: 9090) and remember it
also the other person or client should be told this number
4: Click ok ,something like the image shown below appears
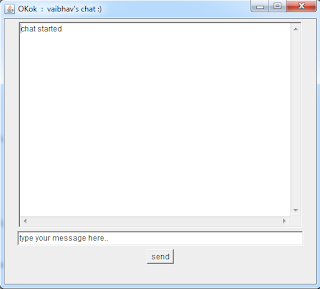
5: Now the person having client.jar should double click it, something like the image shown below will appear
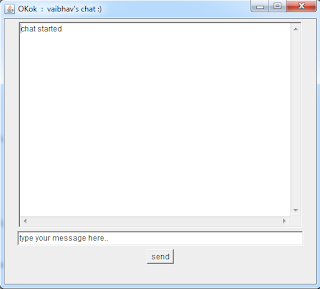
5: Now the person having client.jar should double click it, something like the image shown below will appear
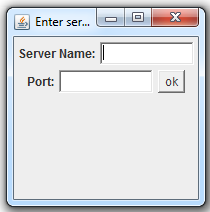
6: Now the client has to enter the IP Address of server in the "server name" field and server's port in the port field
And voila! the client and server can chat now !!
PART 2(The code)
server.java
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.io.*;
import java.net.*;
import javax.swing.*;
public class server
{
public static void main(String[] args)throws IOException
{
Ehandler obj1=new Ehandler(0);//make an object of the class ehandler which implements ActionListener,MouseListener,Runnable and KeyListener
obj1.first();
}
}
class Ehandler implements ActionListener,MouseListener,Runnable,KeyListener
{
String str="chat started";
String temp="";
JFrame mainFrame,popup;
TextArea ta1;
TextField tf1,tf_port;
Button bt_ok;
private ServerSocket ss;
boolean flag=false;
boolean proceed=false;
int port;
public Ehandler(int p)
{
try
{
popup=new JFrame("Enter port");//whenever an object of type Ehandler is created ask for port //number
ta1=new TextArea(str,20,60);
tf1=new TextField("type your message here..",60);
proceed=false;
popup=new JFrame("Enter server name and port");
JLabel l_port=new JLabel("Port:");
tf_port=new TextField(10);
bt_ok =new Button("ok");
bt_ok.addActionListener(this);
popup.setLayout(new FlowLayout());
popup.add(l_port);
popup.add(tf_port);
popup.add(bt_ok);
popup.setSize(200, 200);
popup.setLocation(500, 500);
popup.setVisible(true);
while(!proceed);
popup.setVisible(false);
ss=new ServerSocket(port);
}
catch(IOException e)
{
e.printStackTrace();
}
mainFrame=new JFrame("OKok : vaibhav's chat :)");
}
public void first()
{
Thread t=new Thread(this);
t.start();
tf1.addMouseListener(this);
tf1.addKeyListener(this);
Button b1=new Button("send");
b1.addActionListener(this);
mainFrame.setLayout(new FlowLayout());
mainFrame.getContentPane().add(ta1);
mainFrame.getContentPane().add(tf1);
mainFrame.getContentPane().add(b1);
mainFrame.setSize(500,450);
mainFrame.setLocation(500,200 );
mainFrame.setVisible(true);
}
boolean wait=true;
String cm,m;
public void run()
{
try
{
System.out.println("waiting for client on port "+ss.getLocalPort());
Socket s=ss.accept();
System.out.println("critical");
System.out.println("just connected to "+s.getRemoteSocketAddress());
DataInputStream in=new DataInputStream(s.getInputStream());
DataOutputStream out=new DataOutputStream(s.getOutputStream());
while(!flag)
{
cm=in.readUTF();
str=str+"\nclient:"+cm;
ta1.setText(str);
tf1.setText("enter message..");
wait=true;
while(wait);
System.out.println(m);
out.writeUTF(m);
if((cm.compareTo("bye")==0)||(m.compareTo("bye")==0))
{flag=true;
}
}
s.close();
}
catch(IOException e)
{
System.out.println("exception in run");
}
}
public void actionPerformed(ActionEvent e)
{
if(e.getActionCommand()=="send")
{
sendto();
}
if(e.getActionCommand()=="ok")
{
port=Integer.parseInt(tf_port.getText());
proceed=true;
}
}
public void sendto()
{
System.out.println("send clicked");
String x=tf1.getText();
str=str+ "\nme:"+x;
ta1.setText(str);
tf1.setText("waiting for reply...do not send right now");
m=""+x;
wait=false;
}
public void mouseClicked(MouseEvent e)
{
if(e.getSource()==tf1)
tf1.setText("");
}
public void mouseEntered(MouseEvent e) {}
public void mouseExited(MouseEvent e) {}
public void mousePressed(MouseEvent e) {}
public void mouseReleased(MouseEvent e) {}
public void keyPressed(KeyEvent arg0){}
public void keyReleased(KeyEvent arg0) {}
public void keyTyped(KeyEvent arg0) {
if(arg0.getKeyChar()=='\n')
{
System.out.println("enter pressed");
sendto();
}
}
}
Client.java
import java.io.*;
import java.net.*;
public class client
{
static boolean flag=false;
public static void main(String args[])throws IOException
{
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
System.out.print("enter server name:");
String sname=br.readLine();
System.out.println(sname);
System.out.print("enter port no:");
int port=Integer.parseInt(br.readLine());
try
{
System.out.println("connecting to "+sname+" at port "+port+"..." );
Socket c=new Socket(sname,port);
System.out.println("connected to "+c.getRemoteSocketAddress());
OutputStream o=c.getOutputStream();
DataOutputStream out =new DataOutputStream(o);
String sm;
String message;
while(!flag)
{
System.out.print("enter your message:");
message=br.readLine();
out.writeUTF(message);
if(message.compareTo("bye")==0)
{flag=true;
}
InputStream i=c.getInputStream();
DataInputStream in=new DataInputStream(i);
sm=in.readUTF();
System.out.println("server says:"+sm);
if(sm.compareTo("bye")==0)
{flag=true;
}
}//while
c.close();
}
catch(IOException e)
{
System.out.println("exception occoured !");
}
}
}
Comments
Post a Comment